La cérémonie de remise de matériels agricoles et bureautiques d’un montant d’environ 12 millions FCFA à des coopératives de cacao et au Conseil régional du Cavally s’est déroulée le mercredi 02 avril 2025, à l’esplanade du Conseil régional du Cavally. C’était en présence des autorités administratives, politiques et militaires.
La ministre d’État, ministre de la Fonction Publique, Anne Désirée Ouloto, par ailleurs présidente du Conseil régional du Cavally a été représentée pour la circonstance par le 2e vice-président Youpéhé Marcel qui a salué et félicité les différentes parties prenantes de cet important projet qui vise à encourager l’agroforesterie et à réduire également la pénibilité du travail dans la filière cacaoyère.
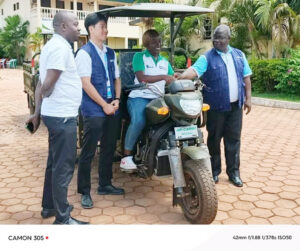
Le matériel distribué est composé de 20 tarières mécanisées pour le planting d’arbres, 20 Kits d’élagages, 20 ébrancheurs motorisés, 50 kits de protection individuels, 8 tricycles pour le transport des produits, 09 ordinateurs portables, des imprimantes, de vidéoprojecteur, d’accessoires informatiques, de kits bureautiques et divers.
Démarré en avril 2023 et lancé officiellement le 22 février 2024, le projet SCOLUR-CI est une initiative du Ministère d’Etat, Ministère de l’Agriculture, du développement rural et des productions vivrières.
Ce projet, financé par le Fonds pour l’environnement mondial (FEM) à hauteur de 5,3 millions USD, ce vise à lutter contre la déforestation et la dégradation des terres en mettant en place des plans de gestion intégrée des paysages cacao-forêts et s’inscrit parfaitement dans le plan national d’investissement agricole de la Côte d’Ivoire (PNIA2) et est mis en œuvre dans l’Indénié-Djuablin, la Mé, le Cavally et le Guémon. Après le Cavally cap a été mis sur le Guémon.
Donatien Zean
- Advertisement -